User guide
Introduction
quantify-scheduler
is a python module for writing quantum programs featuring a hybrid gate-pulse control model with explicit timing control.
It extends the circuit model from quantum information processing by adding a pulse-level representation to operations defined at the gate-level, and the ability to specify timing constraints between operations.
Thus, a user is able to mix gate- and pulse-level operations in a quantum circuit.
In quantify-scheduler
, both a quantum circuit consisting of gates and measurements and a timed sequence of control pulses are described as a Schedule
.
The Schedule
contains information on when operations should be performed.
When adding operations to a schedule, one does not need to specify how to represent this Operation
on all (both gate and pulse) abstraction levels.
Instead, this information can be added later during Compilation.
This allows the user to effortlessly mix the gate- and pulse-level descriptions as is required for many experiments.
We support similar flexibility in the timing constraints, one can either explicitly specify the timing using ScheduleBase.schedulables
, or rely on the compilation which will use the duration of operations to schedule them back-to-back.
Creating a schedule
The most convenient way to interact with a Schedule
is through the quantify_scheduler
API.
In the following example, we will create a function to generate a Schedule
for a Bell experiment and visualize one instance of such a circuit.
# import the Schedule class and some basic operations.
from quantify_scheduler import Schedule
from quantify_scheduler.operations.gate_library import Reset, Measure, CZ, Rxy, X90
def bell_schedule(angles, q0:str, q1:str, repetitions: int):
for acq_index, angle in enumerate(angles):
sched = Schedule(f"Bell experiment on {q0}-{q1}")
sched.add(Reset(q0, q1)) # initialize the qubits
sched.add(X90(qubit=q0))
# Here we use a timing constraint to explicitly schedule the second gate to start
# simultaneously with the first gate.
sched.add(X90(qubit=q1), ref_pt="start", rel_time=0)
sched.add(CZ(qC=q0, qT=q1))
sched.add(Rxy(theta=angle, phi=0, qubit=q0) )
sched.add(Measure(q0, acq_index=acq_index)) # denote where to store the data
sched.add(Measure(q1, acq_index=acq_index), ref_pt="start")
return sched
sched = bell_schedule(
angles=[45.0],
q0="q0",
q1="q1",
repetitions=1024)
# visualize the circuit
f, ax = sched.plot_circuit_diagram()
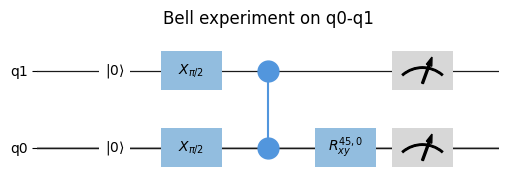
Tip
Creating schedule generating functions is a convenient design pattern when creating measurement code. See the section on execution for an example of how this is used in practice.
Concepts and terminology
quantify-scheduler
can be understood by understanding the following concepts.
Schedule
s describe when an operation needs to be applied.Operation
s describe what needs to be done.Resource
s describe where an operation should be applied.Compilation: between different abstraction layers for execution on physical hardware.
The following table shows an overview of the different concepts and how these are represented at the quantum-circuit layer and quantum-device layer.
Concept |
Quantum-circuit layer |
Quantum-device layer |
|
When |
– |
– |
|
What |
|||
Where |
Quantum-circuit layer
The Quantum-circuit description is an idealized mathematical description of a schedule.
Gates and measurements
In this description operations are quantum gates that act on idealized qubits as part of a quantum circuit.
Operations can be represented by (idealized) unitaries acting on qubits.
The gate_library
contains common operations (including the measurement operation) described at the quantum-circuit level.
The Measure
is a special operation that represents a measurement on a qubit.
In addition to the qubit it acts on, one also needs to specify where to store the data.
Qubits
At the gate-level description, operations are applied to qubits.
Qubits are represented by strings corresponding to the name of a qubit (e.g., q0
, q1
, A1
, QL
, qubit_1
, etc.).
Valid qubits are strings that appear in the device configuration file used when compiling the schedule.
Visualization
A Schedule
containing operations can be visualized using a circuit diagram by calling its method plot_circuit_diagram()
.
Alternatively, one can plot the waveforms in schedules using plot_pulse_diagram()
(the default plotting backend is matplotlib
, but it is possible
to use plotly
by adding the argument plot_backend='plotly'
):
from quantify_scheduler.operations.pulse_library import SquarePulse, RampPulse
from quantify_scheduler.compilation import determine_absolute_timing
schedule = Schedule("waveforms")
schedule.add(SquarePulse(amp=0.2, duration=4e-6, port="P"))
schedule.add(RampPulse(amp=-0.1, offset=.2, duration=6e-6, port="P"))
schedule.add(SquarePulse(amp=0.1, duration=4e-6, port="Q"), ref_pt='start')
determine_absolute_timing(schedule)
_ = schedule.plot_pulse_diagram(sampling_rate=20e6)
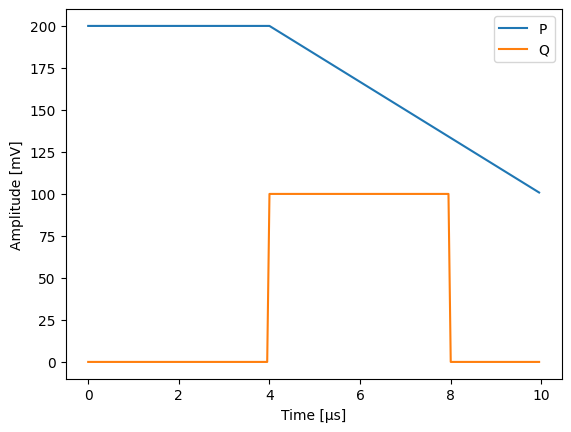
Summary
Gates are described by unitaries.
Gates are applied to qubits.
Measurements are applied to qubits.
Qubits are represented by strings.
Quantum-device layer
The quantum-device layer describes waveforms and acquisition protocols applied to a device. These waveforms can be used to implement the idealized operations expressed on the quantum-circuit layer, or can be used without specifying a corresponding representation at the quantum-circuit layer.
Pulses and acquisition protocols
The pulse-level description typically contains parameterization information, such as amplitudes, durations and so forth required to synthesize the waveform on control hardware.
The pulse_library
contains a collection of commonly used pulses.
Measurements are represented as acquisition protocols.
Acquisition protocols describe the processing steps to perform on an acquired signal in order to interpret it.
The acquisition_library
contains a collection of commonly used acquisition protocols.
Ports and clocks
To specify where an operation is applied, the quantum-device layer description needs to specify both the location in physical space as well as in frequency space.
For many systems, it is possible to associate a qubit with an element or location on a device that a signal can be applied to.
We call such a location on a device a port.
Like qubits, ports are represented as strings (e.g., P0
, feedline_in
, q0:mw_drive
, etc.).
In the last example, a port is associated with a qubit by including the qubit name at the beginning of the port name (separated by a colon :
).
Associating a qubit can be useful when visualizing a schedule and or keeping configuration files readable. It is, however, not required to associate a port with a single qubit. This keeps matters simple when ports are associated with multiple qubits or with non-qubit elements such as tunable couplers.
Besides the physical location on a device, a pulse is typically applied at a certain frequency and with a phase.
These two parameters are stored in a ClockResource
.
Each ClockResource
also has a name
to be easily identified.
The name
should identify the purpose of the clock resource, not the value of the frequency.
By storing the frequency and phase in a clock, we can adjust the frequency of a transition, but refer to it with the same name.
Similar to ports, clocks can be associated with qubits by including the qubit name in the clock name (again, this is not required). If the frequency of a clock is set to 0 (zero), the pulse is applied at baseband and is assumed to be real-valued.
Fig. 1 shows how the resources (qubit, port and clock) map to a physical device.
Fig. 1 Resources are used to indicate where operations are applied.
(a) Ports (purple) indicate a location on a device.
By prefixing the name of a qubit in a port name (separated by a colon :
) a port can be associated with a qubit (red), but this is not required.
(b) Clocks (blue) denote the frequency and phase of a signal.
They can be set to track the phase of a known transition.
By prefixing the name of a qubit in a clock name (separated by a colon :
) a clock can be associated with a qubit (red), but this is not required.
Device image from Dickel (2018) .
Summary
Pulses are described as parameterized waveforms.
Pulses are applied to ports at a frequency specified by a clock.
Ports and clocks are represented by strings.
Acquisition protocols describe the processing steps to perform on an acquired signal in order to interpret it.
Compilation
Different compilation steps are required to go from a high-level description of a schedule to something that can be executed on hardware.
The scheduler supports multiple compilation steps, the most important ones are the step from the quantum-circuit layer (gates) to the quantum-device layer (pulses), and the one from the quantum-device layer into instructions suitable for execution on physical hardware.
The compilation is performed by a QuantifyCompiler
. The compilers are described in detail in Compilers.
This is schematically shown in Fig. 2.
Fig. 2 A schematic overview of the different abstraction layers and the compilation process.
Both a quantum circuit, consisting of gates and measurements of qubits, and timed sequences of control pulses are represented as a Schedule
.
The information specified in the device configuration is used during compilation to add information on how to represent Operation
s specified at the quantum-circuit level as control pulses.
The information in the hardware configuration is then used to compile the control pulses into instructions suitable for hardware execution.
In the first compilation step, pulse information is added to all operations that are not valid pulses (see Operation.valid_pulse
) based on the information specified in the device configuration file.
A second compilation step takes the schedule at the pulse level and translates this for use on a hardware backend. This compilation step is performed using a hardware dependent compiler and uses the information specified in the hardware configuration file.
Note
We use the term “device” to refer to the physical object(s) on the receiving end of the control pulses, e.g. a thin-film chip inside a dilution refrigerator.
And we employ the term “hardware” to refer to the instruments (electronics) that are involved in the pulse generations / signal digitization.
Device configuration
The device configuration is used to compile from the quantum-circuit layer to the quantum-device layer.
The DeviceCompilationConfig
data structure contains the information required to add the quantum-device level representation to every operation in a schedule.
- class DeviceCompilationConfig(**data)[source]
Information required to compile a schedule to the quantum-device layer.
From a point of view of Compilation this information is needed to convert a schedule defined on a quantum-circuit layer to a schedule defined on a quantum-device layer.
Examples
The DeviceCompilationConfig is structured such that it should allow the specification of the circuit-to-device compilation for many different qubit platforms. Here we show a basic configuration for a two-transmon quantum device. In this example, the DeviceCompilationConfig is created by parsing a dictionary containing the relevant information.
Important
Although it is possible to manually create a configuration using dictionaries, this is not recommended. The
QuantumDevice
is responsible for managing and generating configuration files.import pprint from quantify_scheduler.backends.graph_compilation import ( DeviceCompilationConfig ) from quantify_scheduler.schemas.examples.device_example_cfgs import ( example_transmon_cfg ) pprint.pprint(example_transmon_cfg)
{'backend': 'quantify_scheduler.backends.circuit_to_device.compile_circuit_to_device', 'clocks': {'q0.01': 6020000000.0, 'q0.ro': 7040000000.0, 'q1.01': 5020000000.0, 'q1.ro': 6900000000.0}, 'edges': {'q0_q1': {'CZ': {'factory_func': 'quantify_scheduler.operations.pulse_factories.composite_square_pulse', 'factory_kwargs': {'square_amp': 0.5, 'square_clock': 'cl0.baseband', 'square_duration': 2e-08, 'square_port': 'q0:fl', 'virt_z_child_qubit_clock': 'q1.01', 'virt_z_child_qubit_phase': 63, 'virt_z_parent_qubit_clock': 'q0.01', 'virt_z_parent_qubit_phase': 44}}}}, 'elements': {'q0': {'Rxy': {'factory_func': 'quantify_scheduler.operations.pulse_factories.rxy_drag_pulse', 'factory_kwargs': {'amp180': 0.32, 'clock': 'q0.01', 'duration': 2e-08, 'motzoi': 0.45, 'port': 'q0:mw'}, 'gate_info_factory_kwargs': ['theta', 'phi']}, 'Z': {'factory_func': 'quantify_scheduler.operations.pulse_library.SoftSquarePulse', 'factory_kwargs': {'amp': 0.23, 'clock': 'cl0.baseband', 'duration': 4e-09, 'port': 'q0:fl'}}, 'measure': {'factory_func': 'quantify_scheduler.operations.measurement_factories.dispersive_measurement', 'factory_kwargs': {'acq_channel': 0, 'acq_delay': 1.2e-07, 'acq_duration': 3e-07, 'clock': 'q0.ro', 'port': 'q0:res', 'pulse_amp': 0.25, 'pulse_duration': 1.6e-07, 'pulse_type': 'SquarePulse'}, 'gate_info_factory_kwargs': ['acq_index', 'bin_mode', 'acq_protocol']}, 'reset': {'factory_func': 'quantify_scheduler.operations.pulse_library.IdlePulse', 'factory_kwargs': {'duration': 0.0002}}}, 'q1': {'Rxy': {'factory_func': 'quantify_scheduler.operations.pulse_factories.rxy_drag_pulse', 'factory_kwargs': {'amp180': 0.4, 'clock': 'q1.01', 'duration': 2e-08, 'motzoi': 0.25, 'port': 'q1:mw'}, 'gate_info_factory_kwargs': ['theta', 'phi']}, 'measure': {'factory_func': 'quantify_scheduler.operations.measurement_factories.dispersive_measurement', 'factory_kwargs': {'acq_channel': 1, 'acq_delay': 1.2e-07, 'acq_duration': 3e-07, 'clock': 'q1.ro', 'port': 'q1:res', 'pulse_amp': 0.21, 'pulse_duration': 1.6e-07, 'pulse_type': 'SquarePulse'}, 'gate_info_factory_kwargs': ['acq_index', 'bin_mode', 'acq_protocol']}, 'reset': {'factory_func': 'quantify_scheduler.operations.pulse_library.IdlePulse', 'factory_kwargs': {'duration': 0.0002}}}}}
The dictionary can be parsed using the
parse_obj
method.device_cfg = DeviceCompilationConfig.parse_obj(example_transmon_cfg) device_cfg
DeviceCompilationConfig(backend=<function compile_circuit_to_device at 0x7f850f98ba60>, clocks={'q0.01': 6020000000.0, 'q0.ro': 7040000000.0, 'q1.01': 5020000000.0, 'q1.ro': 6900000000.0}, elements={'q0': {'reset': OperationCompilationConfig(factory_func=<class 'quantify_scheduler.operations.pulse_library.IdlePulse'>, factory_kwargs={'duration': 0.0002}, gate_info_factory_kwargs=None), 'Rxy': OperationCompilationConfig(factory_func=<function rxy_drag_pulse at 0x7f850f8ed310>, factory_kwargs={'amp180': 0.32, 'motzoi': 0.45, 'port': 'q0:mw', 'clock': 'q0.01', 'duration': 2e-08}, gate_info_factory_kwargs=['theta', 'phi']), 'Z': OperationCompilationConfig(factory_func=<class 'quantify_scheduler.operations.pulse_library.SoftSquarePulse'>, factory_kwargs={'amp': 0.23, 'duration': 4e-09, 'port': 'q0:fl', 'clock': 'cl0.baseband'}, gate_info_factory_kwargs=None), 'measure': OperationCompilationConfig(factory_func=<function dispersive_measurement at 0x7f850f444160>, factory_kwargs={'port': 'q0:res', 'clock': 'q0.ro', 'pulse_type': 'SquarePulse', 'pulse_amp': 0.25, 'pulse_duration': 1.6e-07, 'acq_delay': 1.2e-07, 'acq_duration': 3e-07, 'acq_channel': 0}, gate_info_factory_kwargs=['acq_index', 'bin_mode', 'acq_protocol'])}, 'q1': {'reset': OperationCompilationConfig(factory_func=<class 'quantify_scheduler.operations.pulse_library.IdlePulse'>, factory_kwargs={'duration': 0.0002}, gate_info_factory_kwargs=None), 'Rxy': OperationCompilationConfig(factory_func=<function rxy_drag_pulse at 0x7f850f8ed310>, factory_kwargs={'amp180': 0.4, 'motzoi': 0.25, 'port': 'q1:mw', 'clock': 'q1.01', 'duration': 2e-08}, gate_info_factory_kwargs=['theta', 'phi']), 'measure': OperationCompilationConfig(factory_func=<function dispersive_measurement at 0x7f850f444160>, factory_kwargs={'port': 'q1:res', 'clock': 'q1.ro', 'pulse_type': 'SquarePulse', 'pulse_amp': 0.21, 'pulse_duration': 1.6e-07, 'acq_delay': 1.2e-07, 'acq_duration': 3e-07, 'acq_channel': 1}, gate_info_factory_kwargs=['acq_index', 'bin_mode', 'acq_protocol'])}}, edges={'q0_q1': {'CZ': OperationCompilationConfig(factory_func=<function composite_square_pulse at 0x7f850f442310>, factory_kwargs={'square_port': 'q0:fl', 'square_clock': 'cl0.baseband', 'square_amp': 0.5, 'square_duration': 2e-08, 'virt_z_parent_qubit_phase': 44, 'virt_z_parent_qubit_clock': 'q0.01', 'virt_z_child_qubit_phase': 63, 'virt_z_child_qubit_clock': 'q1.01'}, gate_info_factory_kwargs=None)}})
Hardware configuration file
The hardware configuration file is used to compile pulses (and acquisition protocols) along with their timing information to instructions compatible with the specific control electronics. To do this, it contains information on what control electronics to compile to and the connectivity: which ports are connected to which hardware outputs/inputs, as well as other hardware-specific settings. Similar to the device configuration file, the hardware configuration file can be written down manually as JSON or be code generated.
Example Qblox hardware configuration file
{
"backend": "quantify_scheduler.backends.qblox_backend.hardware_compile",
"cluster0": {
"ref": "internal",
"instrument_type": "Cluster",
"cluster0_module1": {
"instrument_type": "QCM",
"complex_output_0": {
"lo_name": "lo0",
"portclock_configs": [
{
"port": "q4:mw",
"clock": "q4.01",
"mixer_amp_ratio": 0.9999,
"mixer_phase_error_deg": -4.2
}
]
}
},
"cluster0_module2": {
"instrument_type": "QCM_RF",
"complex_output_0": {
"output_att": 4,
"portclock_configs": [
{
"port": "q0:mw",
"clock": "q0.01"
},
{
"port": "q5:mw",
"clock": "q5.01"
}
]
},
"complex_output_1": {
"output_att": 6,
"portclock_configs": [
{
"port": "q6:mw",
"clock": "q6.01"
}
]
}
},
"cluster0_module3": {
"instrument_type": "QRM",
"complex_output_0": {
"lo_name": "lo1",
"dc_mixer_offset_I": -0.054,
"dc_mixer_offset_Q": -0.034,
"portclock_configs": [
{
"mixer_amp_ratio": 0.9997,
"mixer_phase_error_deg": -4.0,
"port": "q4:res",
"clock": "q4.ro"
}
]
}
},
"cluster0_module4": {
"instrument_type": "QRM_RF",
"complex_input_0": {
"input_att": 10,
"portclock_configs": [
{
"port": "q5:res",
"clock": "q5.ro"
}
]
},
"complex_output_0": {
"output_att": 12,
"portclock_configs": [
{
"port": "q0:res",
"clock": "q0.ro"
}
]
}
},
"cluster0_module10": {
"instrument_type": "QCM",
"real_output_0": {
"portclock_configs": [
{
"port": "q0:fl",
"clock": "cl0.baseband"
}
]
},
"real_output_1": {
"portclock_configs": [
{
"port": "q1:fl",
"clock": "cl0.baseband"
}
]
},
"real_output_2": {
"portclock_configs": [
{
"port": "q2:fl",
"clock": "cl0.baseband"
}
]
},
"real_output_3": {
"portclock_configs": [
{
"port": "q3:fl",
"clock": "cl0.baseband"
}
]
}
},
"cluster0_module12": {
"instrument_type": "QCM",
"real_output_0": {
"portclock_configs": [
{
"port": "q4:fl",
"clock": "cl0.baseband"
}
]
}
}
},
"lo0": {
"instrument_type": "LocalOscillator",
"power": 1
},
"lo1": {
"instrument_type": "LocalOscillator",
"power": 1
}
}
Example Zurich Instruments hardware configuration file
{
"backend": "quantify_scheduler.backends.zhinst_backend.compile_backend",
"mode": "calibration",
"local_oscillators": [
{
"unique_name": "mw_qubit_ch1",
"instrument_name": "mw_qubit",
"frequency_param": "ch1.frequency",
"power": {
"power": 13
}
},
{
"unique_name": "mw_qubit_ch2",
"instrument_name": "mw_qubit",
"frequency_param": "ch2.frequency",
"power": {
"ch_2.power": 10
}
},
{
"unique_name": "mw_readout",
"instrument_name": "mw_readout",
"frequency_param": "frequency",
"power": {
"power": 16
}
}
],
"devices": [
{
"name": "ic_hdawg0",
"type": "HDAWG8",
"clock_select": 0,
"ref": "int",
"channelgrouping": 0,
"channel_0": {
"port": "q0:mw",
"clock": "q0.01",
"mode": "complex",
"local_oscillator": "mw_qubit_ch1",
"markers": [
"AWG_MARKER1",
"AWG_MARKER2"
],
"gain1": 1,
"gain2": 1,
"mixer_corrections": {
"amp_ratio": 0.95,
"phase_error": 0.07,
"dc_offset_I": -0.0542,
"dc_offset_Q": -0.0328
}
},
"channel_1": {
"port": "q1:mw",
"clock": "q1.01",
"mode": "complex",
"local_oscillator": "mw_qubit_ch2",
"markers": [
"AWG_MARKER1",
"AWG_MARKER2"
],
"gain1": 1,
"gain2": 1,
"mixer_corrections": {
"amp_ratio": 0.95,
"phase_error": 0.07,
"dc_offset_I": 0.042,
"dc_offset_Q": 0.028
}
},
"channel_2": {
"port": "q2:mw",
"clock": "q2.01",
"mode": "complex",
"local_oscillator": "mw_qubit_ch2",
"markers": [
"AWG_MARKER1",
"AWG_MARKER2"
],
"gain1": 1,
"gain2": 1,
"mixer_corrections": {
"amp_ratio": 0.95,
"phase_error": 0.07,
"dc_offset_I": 0.042,
"dc_offset_Q": 0.028
}
},
"channel_3": {
"port": "q3:mw",
"clock": "q3.01",
"mode": "complex",
"local_oscillator": "mw_qubit_ch2",
"markers": [
"AWG_MARKER1",
"AWG_MARKER2"
],
"gain1": 1,
"gain2": 1,
"mixer_corrections": {
"amp_ratio": 0.95,
"phase_error": 0.07,
"dc_offset_I": 0.042,
"dc_offset_Q": 0.028
}
}
},
{
"name": "ic_uhfqa0",
"type": "UHFQA",
"ref": "ext",
"channel_0": {
"port": "q0:res",
"clock": "q0.ro",
"mode": "real",
"local_oscillator": "mw_readout",
"trigger": 2
}
}
]
}
Execution
Warning
This section describes functionality that is not fully implemented yet. The documentation describes the intended design and may change as the functionality is added.
Different kinds of instruments
In order to execute a schedule, one needs both physical instruments to execute the compiled instructions as well as a way to manage the calibration parameters used to compile the schedule.
Although one could use manually written configuration files and send the compiled files directly to the hardware, the Quantify framework provides different kinds of Instrument
s to control the experiments and the management of the configuration files (Fig. 3).
Fig. 3 A schematic overview of the different kinds of instruments present in an experiment.
Physical instruments are QCoDeS drivers that are directly responsible for executing commands on the control hardware.
On top of the physical instruments is a hardware abstraction layer, that provides a hardware agnostic interface to execute compiled schedules.
The instruments responsible for experiment control are treated to be as stateless as possible 1 .
The knowledge about the system that is required to generate the configuration files is described by the QuantumDevice
and DeviceElement
s.
Several utility instruments are used to control the flow of the experiments.
Physical instruments
QCoDeS instrument drivers are used to represent the physical hardware. For the purpose of quantify-scheduler, these instruments are treated as stateless, the desired configurations for an experiment being described by the compiled instructions. Because the instruments correspond to physical hardware, there is a significant overhead in querying and configuring these parameters. As such, the state of the instruments in the software is intended to track the state of the physical hardware to facilitate lazy configuration and logging purposes.
Hardware abstraction layer
Because different physical instruments have different interfaces, a hardware abstraction layer serves to provide a uniform interface.
This hardware abstraction layer is implemented as the InstrumentCoordinator
to which individual InstrumentCoordinatorComponent
s are added that provide the uniform interface to the individual instruments.
The quantum device and the device elements
The knowledge of the system is described by the QuantumDevice
and DeviceElement
s.
The QuantumDevice
directly represents the device under test (DUT) and contains a description of the connectivity to the control hardware as well as parameters specifying quantities like cross talk, attenuation and calibrated cable-delays.
The QuantumDevice
also contains references to individual DeviceElement
s, representations of elements on a device (e.g, a transmon qubit) containing the (calibrated) control-pulse parameters.
Because the QuantumDevice
and the DeviceElement
s are an Instrument
, the parameters used to generate the configuration files can be easily managed and are stored in the snapshot containing the experiment’s metadata.
Experiment flow
To use schedules in an experimental setting, in which the parameters used for compilation as well as the schedules themselves routinely change, we provide a framework for performing experiments making use of the concepts of quantify-core
.
Central in this framework are the schedule quantify_scheduler.gettables
that can be used by the quantify_core.measurement.control.MeasurementControl
and are responsible for the experiment flow.
This flow is schematically shown in Fig. 4.
Fig. 4 A schematic overview of the experiments control flow.
Let us consider the example of an experiment used to measure the coherence time \(T_1\). In this experiment, a \(\pi\) pulse is used to excite the qubit, which is left to idle for a time \(\tau\) before it is measured. This experiment is then repeated for different \(\tau\) and averaged.
In terms of settables and gettables to use with the quantify_core.measurement.control.MeasurementControl
, the settable in this experiment is the delay time \(\tau\), and the gettable is the execution of the schedule.
We represent the settable as a qcodes.instrument.parameter.ManualParameter
:
from qcodes.instrument.parameter import ManualParameter
tau = ManualParameter("tau", label=r"Delay time", initial_value=0, unit="s")
To execute the schedule with the right parameters, the ScheduleGettable
needs to have a reference to a template function that generates the schedule, the appropriate keyword arguments for that function, and a reference to the QuantumDevice
to generate the required configuration files.
For the \(T_1\) experiment, quantify-scheduler provides a schedule generating function as part of the quantify_scheduler.schedules.timedomain_schedules
: the quantify_scheduler.schedules.timedomain_schedules.t1_sched()
.
from quantify_scheduler.schedules.timedomain_schedules import t1_sched
schedule_function = t1_sched
Inspecting the quantify_scheduler.schedules.timedomain_schedules.t1_sched()
, we find that we need to provide the times \(\tau\), the name of the qubit, and the number of times we want to repeat the schedule.
Rather than specifying the values of the delay times, we pass the parameter tau
.
qubit_name = "q0"
sched_kwargs = {
"times": tau,
"qubit": qubit_name,
"repetitions": 1024 # could also be a parameter
}
The ScheduleGettable
is set up to evaluate the value of these parameter on every call of ScheduleGettable.get
.
This flexibility allows the user to create template schedules that can then be measured by varying any of it’s input parameters using the quantify_core.measurement.control.MeasurementControl
.
Similar to how the schedule keyword arguments are evaluated for every call to ScheduleGettable.get
, the device config and hardware config files are re-generated from the QuantumDevice
for every iteration.
This ensures that if a calibration parameter is changed on the QuantumDevice
, the compilation will be affected as expected.
from quantify_scheduler.device_under_test.quantum_device import QuantumDevice
device = QuantumDevice(name="quantum_sample")
These ingredients can then be combined to perform the experiment:
from quantify_core.measurement import MeasurementControl
meas_ctrl = MeasurementControl("meas_ctrl")
t1_gettable = ScheduleGettable(
device=device,
schedule_function=schedule_function,
schedule_kwargs=sched_kwargs
)
meas_ctrl.settables(tau)
meas_ctrl.setpoints(times)
meas_ctrl.gettables(t1_gettable)
label = f"T1 experiment {qubit_name}"
dataset = meas_ctrl.run(label)
and the resulting dataset can be analyzed using
# from quantify_core.analysis.t1_analysis import T1Analysis
# analysis = T1Analysis(label=label).run()
Acquisition data format
quantify-scheduler
has multiple interfaces for retrieving acquisition results. This section describes the structure of the return value of the interfaces of InstrumentCoordinator
’s retrieve_acquisition()
and quantify_scheduler.gettables.ScheduleGettable.get()
.
Each acquisition and measurement operation in the schedule has an attached acq_channel
and acq_index
. These can be set through the operation arguments (acq_channel
can optionally be set through subclasses of DeviceElement
, such as BasicTransmonElement
, if the measurement operation is a gate-level operation). Moreover, the bin_mode
parameter can be set explicitly; by default, bin_mode
is average. See the example below:
schedule.add(
SSBIntegrationComplex(
t0=0,
duration=100e-9,
port="q0:res",
clock="q0.ro",
acq_channel=3,
acq_index=1,
bin_mode=BinMode.AVERAGE
)
)
Retrieve acquisitions through InstrumentCoordinator
InstrumentCoordinator
’s retrieve_acquisition()
returns an xarray.Dataset
:
Each
xarray.DataArray
in the dataset corresponds to oneacq_channel
.Each
xarray.DataArray
has two dimensions:acq_index
andrepetition
.
The example below shows the outcome of two binned acquisitions with acquisition channels 0
and 1
and acquisition indices 0
, 1
and 2
for each channel. In this example, repetitions
takes the value of 2
for the schedule and the bin_mode
is set to append. Note that the repetition
dimension only takes on one value (0
) in case bin_mode
is set to average.
<xarray.Dataset> Dimensions: (repetition: 2, acquisition_index: 3) Coordinates: * repetition (repetition) int64 0 1 * acquisition_index (acquisition_index) int64 0 1 2 Data variables: 0 (repetition, acquisition_index) complex128 0j ... (0.1... 1 (repetition, acquisition_index) complex128 0j ... (0.2...
For trace acquisitions, the returned data is still a two-dimensional array: one dimension specifying the repetition, and the other dimension represents the time. Currently it is not possible to use trace acquisition together with append bin_mode
, hence the repetition
dimension will only take one value (0
). See the example below.
<xarray.Dataset> Dimensions: (repetition: 1, acquisition_index: 1000) Coordinates: * repetition (repetition) int64 0 * acquisition_index (acquisition_index) int64 0 1 2 3 4 ... 996 997 998 999 Data variables: 0 (repetition, acquisition_index) complex128 1j 1j ... 1j
Retrieve acquisition through ScheduleGettable
quantify_scheduler.gettables.ScheduleGettable.get()
returns the following data structure for binned acquisitions in the generic case. Note, in the example below real_imag=True
. If this were False
, the result would contain abs
and phase
instead of real
and imag
.
[
# 1st channel
# Real parts
array([
# 1st rep for all indices in channel
real(index0_rep0), real(index1_rep0), real(index2_rep0),
# 2nd rep for all indices in channel
real(index0_rep1), real(index1_rep1), real(index2_rep1),
]),
# Imaginary parts
array([
# 1st rep for all indices in channel
imag(index0_rep0), imag(index1_rep0), imag(index2_rep0),
# 2nd rep for all indices in channel
imag(index0_rep1), imag(index1_rep1), imag(index2_rep1),
]),
# 2nd channel
...
]
For trace acquisition, the generic return value is the following:
[
# Real parts
array([0, 0, 0, 0, ..., 0]),
# Imaginary parts
array([1, 1, 1, 1, ..., 1])
]
Footnotes
- 1
quantify-scheduler
threats physical instruments as stateless in the sense that the compiled instructions contain all information that specifies the execution of a schedule. However, for performance reasons, it is important to not reconfigure all parameters of all instruments whenever a new schedule is executed. The parameters (state) of the instruments are used to track the state of physical instruments to allow lazy configuration as well as ensure metadata containing the current settings is stored correctly.